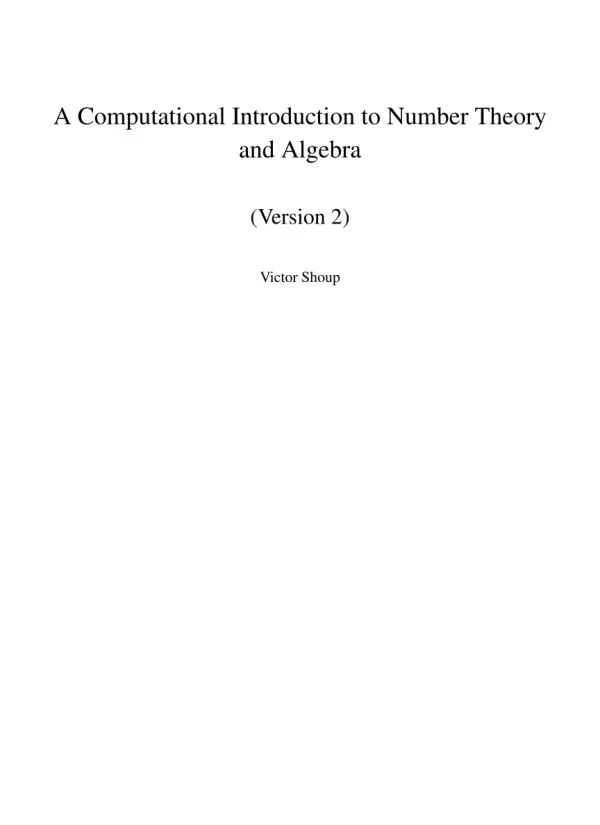
Integer Arithmetic: Theory & Computation
Document information
Author | Victor Shoup |
Major | Number Theory and Algebra |
Document type | Book |
Language | English |
Format | |
Size | 3.48 MB |
Summary
I.Number Theory and Algebra for Computing and Communications Foundational Concepts
This text provides a comprehensive introduction to number theory and abstract algebra, emphasizing algorithms and their applications in cryptography and coding theory. It covers essential topics like unique factorization, congruences, the distribution of primes, groups, rings, fields, and vector spaces. The focus is on commutative structures, providing a simpler and more transparent treatment relevant to the applications discussed. The text also integrates discrete probability theory, crucial for understanding probabilistic algorithms often used in cryptography.
1. Introduction and Scope Number Theory and Algebra in Computing
The preface establishes the book's aim: to offer an accessible introduction to number theory and abstract algebra, focusing on algorithms and applications relevant to computer science and mathematics. It emphasizes the growing importance of these subjects in computing and communications, citing cryptography and coding theory as prime examples. The intended audience is undergraduate or graduate students in computer science or mathematics with some general mathematical background, aiming to bridge the gap between theoretical concepts and practical applications. The author's goal is to make the relevant mathematical topics readily accessible in one place, even if students have prior exposure to some of the material. The text explicitly states that it will develop all necessary mathematics beyond basic calculus from scratch, balancing theoretical explanations with practical applications in a chapter-by-chapter structure. The applications will serve to motivate and illustrate the mathematics, while the mathematical foundations will underpin the applications discussed. This approach acknowledges that a perfect separation of theory and application is unrealistic, and the text will occasionally blend both perspectives. This decision reflects the practical reality of utilizing mathematical concepts to understand and analyze various computing applications. The selection of topics directly reflects their applicability in computing and communications, especially in cryptography and coding theory.
2. Core Mathematical Topics Number Theory and Abstract Algebra
The core mathematical content encompasses foundational elements of number theory and abstract algebra. In number theory, the book covers unique factorization, congruences (including quadratic reciprocity), and the distribution of primes. These form the bedrock for many advanced concepts, particularly in cryptography. In abstract algebra, groups, rings, fields, and vector spaces are explored. The text focuses primarily on commutative structures (abelian groups and commutative rings with unity), a simplification that retains the necessary mathematical tools for addressing the targeted applications while avoiding unnecessary complexities of non-commutative structures. The choice to restrict the scope to commutative structures reflects a deliberate design choice, prioritizing clarity and accessibility for the intended audience without sacrificing the required analytical power for application. The inclusion of discrete probability theory is justified by its necessity in dealing with probabilistic algorithms and cryptographic applications. This interconnectedness showcases how seemingly separate branches of mathematics merge to address real-world computational problems, providing a well-rounded and applicable mathematical framework.
3. Textbook Structure and Approach Balancing Theory and Application
The text's structure is designed to facilitate learning by alternating between theoretical chapters and application-focused chapters. This interleaving method allows students to build a strong mathematical foundation and then immediately see its application in computing. This pedagogy is based on the idea that the theoretical concepts will provide a clear understanding of the underlying principles of the applications, while at the same time, the applications will serve to illustrate and motivate the more abstract mathematical ideas. This approach acknowledges that while striving for a perfect separation between theory and applications is desirable, it is in practice often impossible to maintain this perfect division in the context of computer science. The author makes a deliberate choice to keep the presentation concise and economical, presenting only the absolute minimum of mathematical theory required for a rigorous analysis of the applications discussed. However, the author aims to also develop enough of the relevant theory to provide a well-rounded mathematical narrative and to create a deeper understanding of the mathematical ‘big picture.’ This delicate balance prioritizes efficiency without sacrificing mathematical depth or insight.
II.Efficient Algorithms for Integer Arithmetic
The text details efficient algorithms for basic arithmetic operations on large integers, represented as vectors of base-B digits. It analyzes the complexity of these algorithms (using Big O notation) and stresses the importance of polynomial-time algorithms. Discussions include the trade-offs between implementation in high-level languages versus assembly languages for enhanced performance. Specific algorithms like the repeated-squaring algorithm are covered, showcasing their applications in areas such as computing multiplicative inverses in Zp.
1. Integer Representation and Arithmetic Algorithms
The section begins by defining an idealized computer model, the random access machine (RAM), to provide a precise mathematical framework for analyzing algorithm running times. A key challenge is handling large integers, which may exceed the capacity of a single memory cell. The solution presented is to represent these large integers as vectors of digits in a fixed base. Each digit is chosen to fit within a single memory cell, thus addressing the limitation of memory capacity. The choice of base B is a critical consideration; it's suggested to use a base roughly half the machine's word size to prevent overflow. However, using assembly language allows a base much closer to, or equal to, the word size, leading to significant performance gains. The text emphasizes that efficient algorithms for large integers should have running times that are polynomial in the bit lengths of the inputs, not their magnitudes. This distinction is crucial, as algorithms with running times dependent on the magnitude of inputs can quickly become impractical even for moderately sized inputs. The difference between polynomial time (e.g., O(l^2), where 'l' is the bit length) and non-polynomial time (e.g., O(n^(1/2)), where 'n' is the magnitude) is highlighted, emphasizing the significant increase in computational time as input size grows. Efficient algorithms are paramount in the field, a point underscored by using the function len(a) instead of log a in Big O notation; this clarifies the reliance on bit length.
2. Algorithm Analysis and Big O Notation
The text uses Big O notation to express the running times of algorithms, abstracting away implementation-specific constant factors. This approach offers a generalized measure of an algorithm's efficiency that remains consistent across different implementations or machine architectures. The discussion highlights the trade-offs inherent in algorithm design, particularly the balance between simplicity and efficiency. For instance, while a brute-force approach (like trying all possibilities in a base-B conversion) might be conceptually straightforward, more sophisticated algorithms are often required to achieve better runtime performance. This choice is influenced not only by practical considerations but also by theoretical limits; an algorithm's complexity class provides crucial insight into its scalability. The text notes that the choice of notation, specifically len(a)
over log a
is intentional, emphasizing that algorithm performance is linked to the bit-length of the inputs. The use of len(a) avoids issues that may occur with functions like log which can become undefined or vanish for certain inputs. This methodological detail reflects a commitment to rigorous analysis and a transparent explanation of the chosen methods.
3. Practical Implementations and Efficiency Considerations
The discussion expands upon practical considerations in implementing algorithms for integer arithmetic. The text observes that the most efficient implementations frequently involve low-level assembly language programming, tailored to specific machine architectures. This approach offers advantages beyond the general efficiency improvements over compiler-generated code; it allows direct access to low-level machine instructions (such as those supporting 64-bit multiplication of 32-bit integers) unavailable in higher-level languages. This provides considerable performance gains; the example of doubling the bit-length of B resulting in a fourfold decrease in the running time of multiplication and division algorithms illustrates this point. This potential for substantial speed-ups (five- to ten-fold compared to higher-level language implementations) highlights the importance of efficient implementation techniques for computationally demanding tasks such as those often found in cryptography and number theory. The section subtly contrasts the idealized RAM model of computation with real-world limitations, underscoring the practical relevance of the discussed algorithms. The text uses this as a motivation to encourage readers to consider the practical implementations of such efficient algorithms.
III.The Extended Euclidean Algorithm and its Applications
This section delves into the extended Euclidean algorithm, demonstrating its use in solving problems such as Thue's lemma and rational reconstruction. The importance of these techniques for efficient computations in number theory is highlighted, including applications in solving linear equations with rational solutions and in more advanced algorithms. The algorithm's efficiency is analyzed using complexity measures such as Big O notation.
1. The Extended Euclidean Algorithm and Thue s Lemma
A key focus is the application of the extended Euclidean algorithm to provide an efficient solution to Thue's Lemma. The text highlights that while Thue's Lemma guarantees the existence of certain numbers, the 'pigeonhole principle' proof doesn't directly translate to an efficient algorithm for finding them. The extended Euclidean algorithm is presented as a more practical method to obtain these numbers as a 'natural byproduct.' This observation is important because it converts a purely existential mathematical result into an effective computational procedure. The efficiency gains from using the extended Euclidean algorithm are significant because it allows for the computation of values that would otherwise be difficult to find using a naive approach, directly demonstrating the practical value of this algorithm within the computational context of number theory. The approach enhances the computational feasibility of problems relying on Thue's Lemma, transforming a theoretical guarantee into a practical tool for algorithmic problem solving. The algorithm's computational efficiency is a crucial aspect emphasized throughout the section, underlining its significance within the framework of efficient computational number theory.
2. Rational Reconstruction and its Algorithmic Solution
The concept of rational reconstruction is introduced as a problem arising from the application of the extended Euclidean algorithm. The problem is defined as follows: given integers n, b, r*, t* (with specific conditions), find integers r and t such that r/t satisfies a given equation, using r0 and t0 found via the extended Euclidean algorithm. This approach effectively utilizes the extended Euclidean algorithm to solve the rational reconstruction problem efficiently. The method's efficiency is highlighted by its requirement of only O(len(M)) digits, where M is a relevant parameter. This efficiency consideration is crucial in determining the practicality of utilizing the technique for large numbers. The efficiency of this approach makes it especially useful in contexts where computational efficiency is paramount. This section emphasizes the practical applicability and efficient solvability of the rational reconstruction problem, utilizing the extended Euclidean algorithm for efficient computation in number theory.
3. Applications in Symbolic Algebra and Beyond
The section briefly discusses the applications of rational reconstruction in symbolic algebra. The specific example presented involves solving the equation vA = w, where A is a non-singular square integer matrix and w is an integer vector. The solution vector v will generally contain rational entries. The algorithm using rational reconstruction directly computes the exact solution rather than resorting to floating-point approximations, making it a precise tool for symbolic computation. The text contrasts this method with other approaches like Gaussian elimination, which could potentially lead to large numerators and denominators in intermediate computations, potentially impacting the efficiency of the overall computation. While Gaussian elimination can still provide a solution in polynomial time, the use of rational reconstruction is shown to offer potential efficiency benefits, particularly in scenarios where computational cost is a major concern. This makes it a valuable tool for symbolic algebra and computation.
IV.The RSA Cryptosystem and its Security Considerations
The text introduces the RSA cryptosystem, a pivotal application of number theory in cryptography. It explains the system's operation and discusses various security considerations, emphasizing the vulnerability of deterministic encryption and the need for probabilistic algorithms to mitigate attacks. The relationship between the difficulty of factoring large numbers and the security of the RSA system is explored. The section emphasizes the role of number theory and abstract algebra in the secure implementation of RSA.
1. Overview of the RSA Cryptosystem
This section provides an overview of the RSA cryptosystem, named after its inventors Rivest, Shamir, and Adleman. The text emphasizes that while the basic operation of the system can be understood with the tools presented, a complete understanding requires further concepts developed later in the book. This acknowledgment highlights the layered nature of the subject matter and the necessity of a foundational understanding of number theory and algebra for a deeper grasp of cryptography. The introduction of RSA at this point in the book is deliberate, serving as an early demonstration of the practical applications of the mathematical concepts being introduced. This strategy motivates the learner by immediately showing the real-world relevance of the mathematical material, linking abstract concepts to tangible cryptographic applications. The choice to introduce RSA early reflects a pedagogical decision prioritizing motivation over a purely sequential presentation of mathematical theory.
2. Security Concerns and Attacks on RSA
The section warns that the security of encryption schemes is a complex topic beyond the scope of the text. It acknowledges limitations of the simple RSA version described, noting that it's vulnerable to certain attacks due to the deterministic nature of the encryption algorithm. A specific example is given: if an adversary knows the set of possible messages, they can encrypt each one and compare to the intercepted ciphertext to determine the original message. This vulnerability underscores the need for probabilistic encryption algorithms. The text suggests using random bit padding to enhance RSA's security but also cautions that this must be done carefully. This discussion highlights that simply assuming the infeasibility of factoring large numbers—often cited as the basis for RSA's security—is insufficient to guarantee the system's invulnerability. Even if factoring is computationally intractable, the possibility of other, less obvious attacks exists, underscoring the ongoing challenges of cryptographic security and the need for a comprehensive understanding of both the underlying mathematics and the potential weaknesses of implemented systems.
3. Mitigation Strategies and Further Considerations
The text mentions that computing the decryption exponent (d) in RSA is computationally equivalent to factoring n (the modulus), which is currently believed to be infeasible for suitably large n. This observation is important for the security of the system. However, the text notes that this doesn't fully guarantee security, as other attacks might still exist. This nuanced perspective reflects the ongoing research and evolution of cryptographic security. It also emphasizes the need to go beyond simply asserting the computational difficulty of factoring to consider a more holistic range of potential vulnerabilities. The discussion serves as a cautionary note for anyone relying on the RSA cryptosystem, highlighting the subtleties involved in ensuring cryptographic security and the importance of ongoing research to mitigate evolving threats. The text doesn't provide exhaustive security proofs but provides a crucial foundation for further understanding the security complexities inherent in RSA and public-key cryptography in general.
V.Distribution of Primes and the Prime Number Theorem
This section explores the distribution of prime numbers. It introduces the prime number theorem and related concepts, such as Chebyshev's theta function and the Riemann hypothesis. While not directly used in subsequent chapters, this overview broadens the reader's perspective on the rich mathematical landscape related to primes. The section touches on important mathematical results and conjectures regarding prime number distribution.
1. Overview of Prime Number Distribution
This section provides a broad overview of theorems and conjectures related to the distribution of prime numbers, acknowledging that this is a vast area of mathematical research. The text states that several theorems will be presented without proof, aiming for self-containment but also recognizing the value of providing a broader perspective. This approach reflects a balance between providing sufficient detail for understanding the core concepts and acknowledging the depth and complexity of the broader subject. The section's purpose is to offer context and a wider view of the mathematical landscape surrounding prime numbers, enriching the reader's understanding without delving too deeply into complex proofs that would be beyond the scope of this introductory text. The stated intention is to avoid excessive mathematical detail while still conveying important ideas related to prime distribution, maintaining a suitable level of comprehensibility for the intended audience.
2. The Prime Number Theorem and Related Conjectures
The section introduces the prime number theorem and discusses related theorems and conjectures, notably the Riemann Hypothesis. The Riemann Hypothesis, presented as a conjecture, is described as one of the most challenging unsolved problems in mathematics. The text notes the connection between the Riemann Hypothesis and sharper estimates of the prime-counting function, π(x). This is significant because it highlights the profound implications that this conjecture would have on our understanding of prime distribution. The authors include a cautionary note about differing definitions of the logarithmic integral, highlighting a potential source of confusion stemming from variations in the mathematical literature. The inclusion of historical notes, mentioning the work of Dirichlet (1837) and de la Vallée Poussin (1896), adds context and emphasizes the rich history of research in this area. References to other works and theorems (Oesterlé, Heath-Brown) further demonstrate the extensive body of work surrounding prime distribution.
3. Historical Context and Further Reading
The concluding notes of the section provide historical context and suggest further reading on the topic. It mentions Euler's 18th-century work establishing a connection between prime numbers and the zeta function, a fundamental result in the history of number theory. The Riemann Hypothesis, proposed by Riemann in 1859, is again highlighted for its importance and continuing impact on the field. The text notes that Riemann showed the equivalence of his conjecture to specific estimates of π(x). Later work by von Koch (1901) further refined this connection. This historical context frames the discussion in a broader mathematical history, demonstrating the deep and continuing investigation into prime number distribution. References to Crandall and Pomerance's book [30] and specific exercises within that book encourage readers to delve further into this area of number theory. This approach encourages further learning while maintaining a focus on providing a foundational and accessible introduction within this text.